- Python -m unittest runner or python runner.py. Triangles on November 08, 2019 at 11:10 @Manohar runner.py is a regular script, so `python runner.py` should be enough.
- One more question: do you know why unittest.main would return zero test (hence it should not be run), when the module is run as a test file (using python -m unittest testcities.py as you mentioned)? I understand that running it this way will make the name to become the module name (testcities), hence the need to use if name.
- Python Unittest Run Tests In Order
- Python Unittest Run Single Test
- Python Run Unittest Command Line
- Python Unit Test Runner Gui
- Unit Testing With Python
- How To Test Python Code
Why to unit test your python source code?
Python testing in Visual Studio Code. The Python extension supports testing with Python's built-in unittest framework as well as pytest. A little background on unit testing (If you're already familiar with unit testing, you can skip to the walkthroughs.) A unit is a specific piece of code to be tested, such as a function or a class. +1 and since terminology can be confusing if new to a language (and the usage is even oddly inconsistent): running python -m unittest moduletest.TestClass.testmethod assumes a file moduletest.py (run from current directory; and init.py is not required); and moduletest.py contains class TestClass(unittest.TestCase). Which contains def testmethod(self.) (this also works for me on.
All programmers want their code to be impeccable, but as the saying goes, to err is human, we make mistakes and leave bugs in our source code. Here is where the unit testing comes to our rescue. If you use unit testing from the very beginning of your code development, it will be easier to detect and remove the bugs not only at the beginning stage of development but also post-production.
Unit tests also make us comfortable while refactoring and help to find any bugs left due to an update in the source code. If you are looking for a career in python development, then unit testing your source code is a must for all the big companies. So let us dive into the testing.
Unittest module
Since I have convinced you to use the unit testing with your python source codes, I will illustrate the process in detail. There are various test-runners in python like unittest, nose/nose2, pytest, etc. We will use unittest to test our python source code. The unittest is an inbuilt module and using it is as easy as:-
Writing a simple unit test in python
Let us assume, we have the following python code:-
We will create a new file for the test cases of the code. The general convention is to use either test_filename.py or filename_test.py. We will use test_filename.py. We will keep both the files in the same directory to make the imports and usage relatively easier.
Code explanation:-
- We imported the unittest module and calculator.
- Created a class inheriting from unittest.TestCase().
- Then we defined our test for the addition function. You must note that the method/function must start with test_. Otherwise, it won’t run. See the example below:-
- Finally, we have used the assertEqual statement. You can get the list of the assert statements from here and use it as per your case. Few common assert statements are as under:-
Method | Checks for |
---|---|
assertEqual(a, b) | a b |
assertNotEqual(a, b) | a != b |
assertTrue(x) | bool(x) is True |
assertFalse(x) | bool(x) is False |
assertIs(a, b) | a is b |
assertIsNot(a, b) | a is not b |
assertIsNone(x) | x is None |
assertIsNotNone(x) | x is not None |
assertIn(a, b) | a in b |
assertNotIn(a, b) | a not in b |
assertIsInstance(a, b) | isinstance(a, b) |
assertNotIsInstance(a, b) | not isinstance(a, b) |
Now there are three ways to run the test:-
- You can run it from the terminal using the code:-
python -m unittest test_calculator.py
- You can also run it from the terminal using the code:-
python -m unittest
This will automatically detect all the unit tests and run them. The downside of it is that if you have multiple files and tests, it will run all of them. - The last and my favorite method is to use the dunders method:
Then you can run the test using the code:-python test_calculator.py
The advantage of using this method is that you can run the test from the text-editor as well.
Running the above test will give us the following output:-
It has run one test, and the test is OK (passed).
If there is some error in our code, e.g. in multiplication if we have mistyped ‘**‘ instead of ‘*‘. Then running the test will give us the error.
The output will be :-
Understanding the test outputs
The test output will have the following parts:-
1. The first line will show the summary result of the execution of all the tests. “.” - test passed and “F” - test failed.
2. If all the tests have passed, the next line will show the number of tests and time taken followed by “OK” in the following line.
3. If any/all the tests fail, the second line will show the name of the test failed, followed by traceback.
4. The error will be raised in the next line.
5. Next line will show the number of tests ran and time taken.
6. The last line will show ‘FAILED’ and the number of failures.
You can also pass your own, AssertionError message as under:-
Handling raised error with unittest
In the above example, we have raised a value error in the divide() function. We need to test that divide by zero will raise the error correctly.
We will use assertRaises with the context manager and create the following test in our class TestCalculator():-
If we will run the test:-
”.” shows that our test has passed. It means that our program will raise a value error when the number is divided by 0.
Using unittest module in a more complex example
We will use the unittest in a more complex example. For that, I downloaded the following code from Corey Schafer’s OOP tutorial.
Now, when we will create an instance of the employee with the first and the last name, it will automatically create the email and full name of the employee. Also, on changing the first or the last name of the employee should change the email and full name. To test the same, we will create the following tests
Running this will give the following output:-
Two ‘.’ show that the tests have passed.
Using setUp and tearDown methods in unittest
In the above test, we are creating the instances of the individual for each test and hence violating the ‘DRY’ convention. To overcome this problem, we can use setUp and tearDown methods and change our code as under. For now, we are simply passing the tearDown method, but it is useful when the testing involves creating files, databases etc. and we want to delete them at the end of each test and start with a clean slate. For better a illustration of how it works, we will add print() function in our tests. Offline bluestack app player setup file (. msi).
Output:-
The output depicts that the setUp function ran before every test and tearDown function ran after every test. This could be useful if you are running multiple tests.
Python Unittest Run Tests In Order
In some of the use-cases, it might be useful to have some code run before the whole set of unit tests and something at the end of the unit tests. In such a scenario, you can use two class methods named setUpClass and tearDownClass.
Test-driven development
In the above examples, we have developed/written the code and thereafter written the tests for that code. However, many developers will write the tests first and then code. This is called “test-driven development” and is very popular among pro developers.
Assume that you are asked to write a program to find the area of a circle. The easiest function to write is as under:-
It seems fine, but now try running it as under:-
The output of this will be:-
Surprised? So, the above easy looking function has calculated the area for a positive, negative, complex number and boolean radii. Now, let us proceed with test-driven development and start writing the tests:-
We created a test for radius >=0 and used assertAlmostEqual to assert the value. This test will pass. Now we will integrate the following two cases in our tests:-
1. The function should raise a ValueError for negative radius.
2. The function should raise a TypeError for the radius of a type other than integer and float.
Running this test will give us the following output:-
Gta vice city 5 game free. download full version pc. So, one of our tests has passed and rest has failed with the following Assertionerrors:-
1. AssertionError: TypeError not raised
2. AssertionError: ValueError not raised
The test output shows to raise specific errors. Now let us change our code as under:-
Since we have raised the TypeError and ValueError in our code, the tests will pass.
Using mocking with unittest for web requests
There are few situations in which we don’t have any control e.g. if we are doing web-scrapping where our function goes to a website and get some information from it. If the website is down, our function will fail but that will also result in our tests getting failed. However, we want our test to fail only when there is some error in our code. We will use mocking to overcome this problem. Let us have a look at the following example:-
The test for checking this code will be as under:-
- Here, we have used patch from unittest.mock() and have run it as a context manager.
- Then, if the response is “Ok”, we have set the text as “Success” and then used assertEqual.
- If the website is down, then there will get ‘Bad Response’.
The output of the test is:-
I will wrap up this tutorial with a hope that you will be comfortable in unit testing your python source code.
In case of any query, you can leave the comment below.
If you liked our tutorial, there are various ways to support us, the easiest is to share this post. You can also follow us on facebook, twitter and youtube.
If you want to support our work. You can do it using Patreon.
Latest versionReleased:
Parallel unit test runner with coverage support
Project description
unittest-parallel is a parallel unit test runner for Python with coverage support.
To run unittest-parallel, specify the directory containing your unit tests with the '-s' argumentand your package's top-level directory using the '-t' argument:
By default, unittest-parallel runs unit tests on all CPU cores available.
To run your unit tests with coverage, add either the '--coverage' option (for line coverage) or the'--coverage-branch' for line and branch coverage.
How it works
unittest-parallel uses Python's built-in unit test discovery to find all test cases in your project.It then runs all test cases in a Python multi-processing pool of the requested size.
Class and Module Fixtures
Python's unittest framework supportsclass and module fixtures.Use the '--class-fixtures' option to execute class fixtures correctly. Use the '--module-fixtures'option to execute module fixtures correctly. Note that these options reduce the amount ofparallelism.
Example output
Usage
Development
This project is developed using python-build. It was startedusing python-template as follows:
Release historyRelease notifications | RSS feed
1.5.0
1.4.6
1.4.5
1.4.4
1.4.3
1.4.1
1.4.0
1.3.1
1.3.0
1.2.1
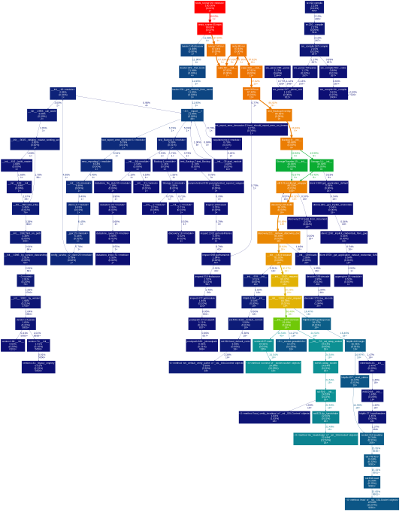
Python Unittest Run Single Test
1.2.0
1.1.3
1.1.2
1.1.1
1.1.0
1.0.7
Python Run Unittest Command Line
1.0.6
1.0.5
1.0.4
1.0.3
1.0.2
1.0.1
1.0
0.8.7
0.8.2
Python Unit Test Runner Gui
0.8.1
Unit Testing With Python
0.8
0.5
0.4
How To Test Python Code
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size unittest-parallel-1.5.0.tar.gz (9.2 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for unittest-parallel-1.5.0.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | 53ac8c10d148a61de0faca8cbc00e73c942a29e4fb96e2d34283ee2365a5697b |
MD5 | 60be848068290c02f4685eca4b65fa61 |
BLAKE2-256 | 3dcc98a2438a9522cc99dd2954565173887f250647dfcbf1e3ce8a0fec8c14ce |
Comments are closed.